Vue js format numbers as currency strings:To format numbers as currency strings in Vue.js using native JavaScript, the toLocaleString() method can be employed. This method, accessible through JavaScript’s Number prototype, permits the formatting of numbers based on the user’s locale.
How can I format numbers as currency strings in Vue.js using the currency format used in the United States of America (USA)?
In Vue.js, you can format numbers as currency strings using the toLocaleString
method. In the given code snippet, a computed property named formattedPrice
is defined. Inside this property, the toLocaleString
method is used to format the price
variable as a currency string in the USA (en-US) locale with the currency set to USD.
The style
option is set to 'currency'
, which indicates that the formatting should be done as currency
Vue js format numbers as (USA) currency strings Example
<div id="app">
<p>price: {{price}}</p>
<p>format Price: {{ formattedPrice }}</p>
</div>
<script>
const app = new Vue({
el: "#app",
data() {
return {
price: 1234.56
};
},
computed: {
formattedPrice() {
// Format the price using the toLocaleString method with en-US locale and USD currency
return this.price.toLocaleString('en-US', { style: 'currency', currency: 'USD' });
}
}
});
</script>
Output of Vue js format numbers as currency strings
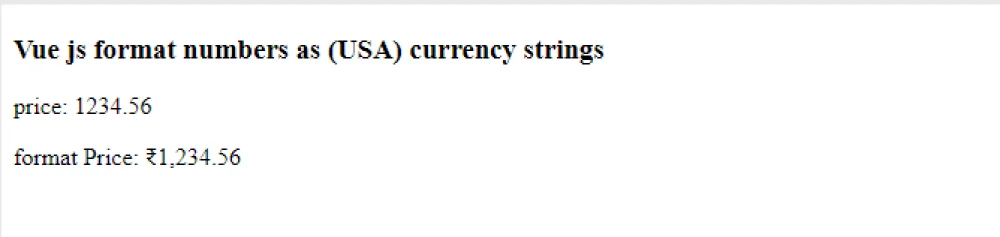
How to format numbers as Chinese currency strings (CNY) in Vue.js?
The formattedPrice()
function in Vue.js formats numbers as Chinese currency strings (CNY) using the toLocaleString
method. It takes the price
value and applies the toLocaleString
method with the zh-CN
locale and CNY
currency options.
This method formats the number as a currency string in the Chinese format, including the currency symbol (CNY) and appropriate separators for thousands and decimals.
The formatted price is then returned by the function. This allows for easy display of prices in a user-friendly and culturally appropriate format for Chinese users.
Vue js format numbers as (CNY) currency strings Example
<div id="app">
<p>price: {{price}}</p>
<p>Format Price: {{ formattedPrice }}</p>
</div>
<script>
const app = new Vue({
el: "#app",
data() {
return {
price: 4567.51
};
},
computed: {
formattedPrice() {
// Format the price using the toLocaleString method with zn-cn locale and CNY currency
return this.price.toLocaleString('zh-CN', { style: 'currency', currency: 'CNY' });
}
}
});
</script>
Output of Vue js format numbers as (CNY) currency strings

How to Format currency strings in Vue.js for Indian Rupees (INR) display?
The formattedPrice()
function in Vue.js is used to format currency strings specifically for displaying Indian Rupees (INR). It utilizes the toLocaleString
method with the ‘en-IN’ locale and ‘INR’ currency options.
This method converts the numeric value stored in this.price
to a localized string representation, following the formatting rules of the ‘en-IN’ locale. It applies the currency style and uses the ‘INR’ currency symbol.
The formatted price string is then returned by the function, ready for display in the desired currency format for Indian Rupees.
Vue Js format (INR) currency strings Example
<div id="app">
<p>price: {{price}}</p>
<p>Format Price: {{ formattedPrice }}</p>
</div>
<script>
const app = new Vue({
el: "#app",
data() {
return {
price: 234234.45
};
},
computed: {
formattedPrice() {
// Format the price using the toLocaleString method with en-IN locale and INR currency
return this.price.toLocaleString('en-IN', { style: 'currency', currency: 'INR' });
}
}
});
</script>
Output of Vue Js format (INR) currency strings
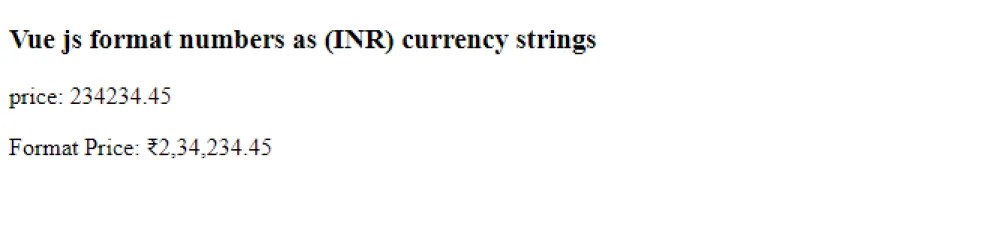
How to format numbers as (EUR) currency strings in Vue.js?
The function formattedPrice()
formats a given number, this.price
, as a currency string in Euro (EUR) format. It achieves this by utilizing the toLocaleString
method with the ‘it-IT’ locale and ‘EUR’ currency.
This ensures that the number is formatted according to Italian conventions for currency representation, including the appropriate currency symbol (€) and decimal separators.
Vue js format numbers as (EUR) currency strings Example
<div id="app">
<p>price: {{price}}</p>
<p>Format Price: {{ formattedPrice }}</p>
</div>
<script>
const app = new Vue({
el: "#app",
data() {
return {
price: 23224.45
};
},
computed: {
formattedPrice() {
// Format the price using the toLocaleString method with it-IT locale and EURf currency
return this.price.toLocaleString('it-IT', { style: 'currency', currency: 'EUR' });
}
}
});
</script>
Output of Vue js format numbers as (EUR) currency strings
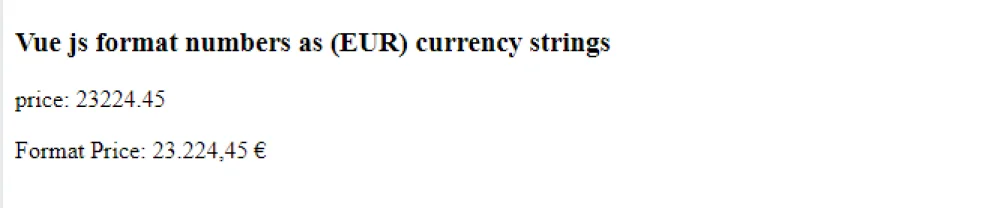